In this interesting post, we will explore two terms we often hear from data scientists i.e. Extrapolation & Interpolation. When we get access to data points, we try to present all the information that can be useful.
Let’s start exploring this bit from the AI universe.
Interpolation vs Extrapolation
The prefix inter- means between, and extra- means beyond.
Interpolation is a type of estimation, a method of constructing new data points within the range of a discrete set of known data points.
Extrapolation is a type of estimation, beyond the original observation range, the value of a variable on the basis of its relationship with another variable.
Interpolation vs Extrapolation: Graphical Representation
Let’s consider a few points.
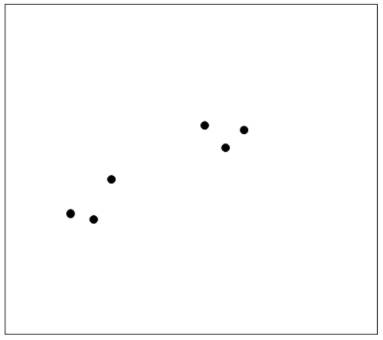
Fit a straight line(we can also fit fancy mathematical functions, just for simplicity we have considered fitting a straight line) in a way that generalizes all the points.
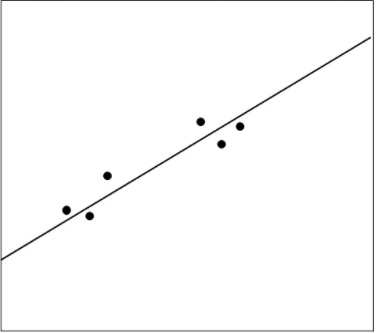
The line lets you both interpolate (generate expected values in between your data points) and extrapolate (generate expected values outside the range of your data points).
Interpolation is guessing data points that fall within the range of the data you have, i.e. between your existing data points. Extrapolation is guessing data points from beyond the range of your known data set.
An Interesting Example of Interpolation and Extrapolation
If I tell you that I had 7 dollars the day before yesterday, and 3 dollars left today, you can interpolate that I had 5 dollars yesterday. You could also extrapolate that I will have 1 dollar tomorrow. You could extrapolate that I will have -1 dollar the day after tomorrow, illustrating one of the many pitfalls of extrapolation.
Think over this pitfall.
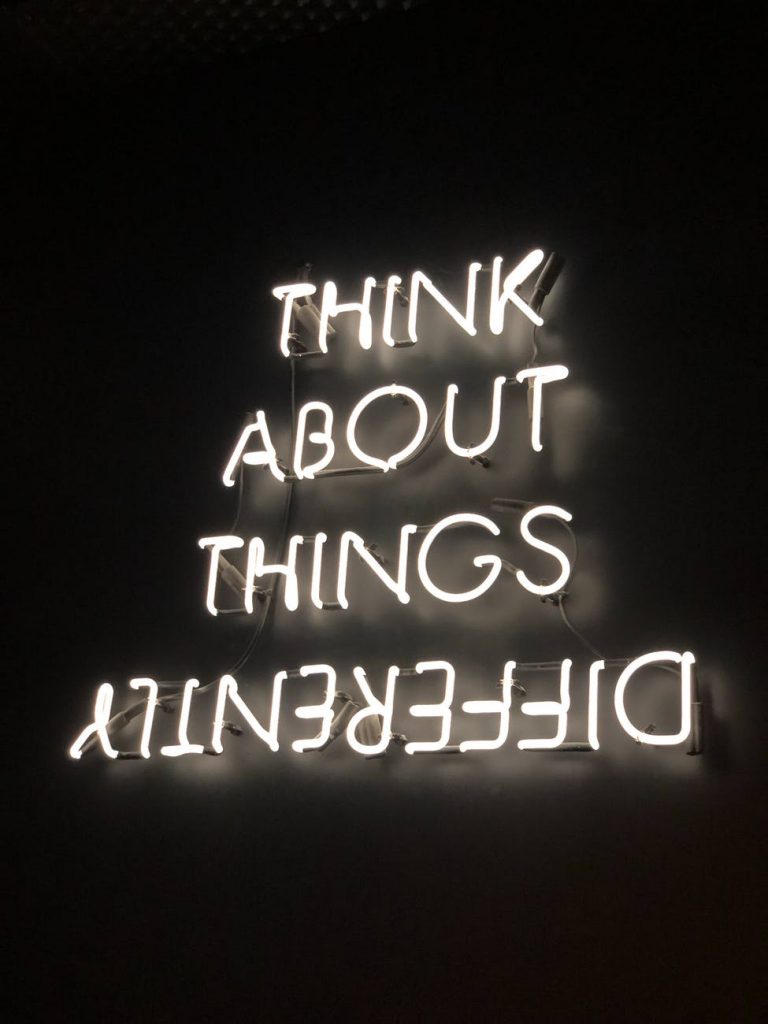
It points towards a risk that extrapolation is subjected to greater uncertainty and a higher risk of producing meaningless results.
Common interpolation methods: Linear Interpolation, Polynomial Interpolation & Spline Interpolation with Python Code
Interpolation is a technique used to estimate values between two known values. It is commonly used in data analysis, numerical analysis, and computer graphics. There are several interpolation methods available, but here are three of the most popular ones:
Linear Interpolation with Python Code
What is Linear Interpolation?
Linear Interpolation is a simple method that assumes a linear relationship between two known data points. It connects the two points with a straight line and estimates values between them. It is a good choice for data that changes linearly.
Python Code for Linear Interpolation
To implement linear interpolation in Python, you can use the interp1d
function from the scipy.interpolate
module:
import numpy as np
from scipy.interpolate import interp1d
# Example data
x = np.array([0, 1, 2, 3])
y = np.array([0, 2, 4, 6])
# Linear interpolation function
f = interp1d(x, y)
# Estimate values
x_new = np.linspace(0, 3, num=10)
y_new = f(x_new)
# Plot original data and estimated values
import matplotlib.pyplot as plt
plt.plot(x, y, 'o', label='data')
plt.plot(x_new, y_new, '-', label='linear')
plt.legend()
plt.show()
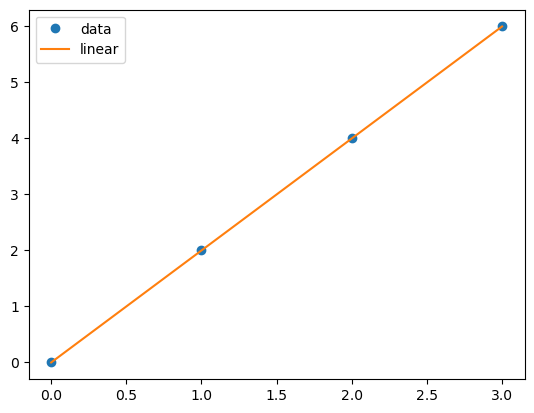
Polynomial Interpolation with Python Code
What is Polynomial Interpolation?
Polynomial interpolation is a method that fits a polynomial curve to the data points. It is a good choice for data that has a non-linear relationship between two points.
Python Code for Polynomial Interpolation
To implement polynomial interpolation in Python, you can use the polyfit
function from the numpy
module:
import numpy as np
# Example data
x = np.array([0, 1, 2, 3])
y = np.array([0, 1, 4, 9])
# Polynomial interpolation function
p = np.polyfit(x, y, deg=2)
f = np.poly1d(p)
# Estimate values
x_new = np.linspace(0, 3, num=10)
y_new = f(x_new)
# Plot original data and estimated values
import matplotlib.pyplot as plt
plt.plot(x, y, 'o', label='data')
plt.plot(x_new, y_new, '-', label='polynomial')
plt.legend()
plt.show()
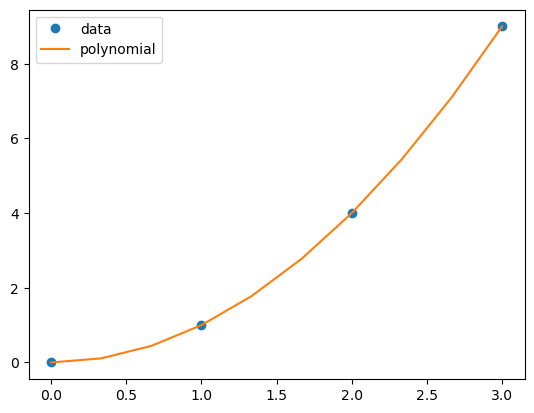
Spline Interpolation with Python Code
What is Spline Interpolation?
Spline interpolation is a method that fits a piecewise polynomial curve to the data points. It is a good choice for data that has a complex or erratic relationship between two points.
Python Code for Spline Interpolation
To implement spline interpolation in Python, you can use the splrep
and splev
functions from the scipy.interpolate
module:
import numpy as np
from scipy.interpolate import splrep, splev
# Example data
x = np.array([0, 1, 2, 3])
y = np.array([0, 2, 4, 6])
# Spline interpolation function
spl = splrep(x, y)
f = splev(x, spl)
# Estimate values
x_new = np.linspace(0, 3, num=10)
y_new = splev(x_new, spl)
# Plot original data and estimated values
import matplotlib.pyplot as plt
plt.plot(x, y, 'o', label='data')
plt.plot(x_new, y_new, '-', label='spline')
plt.legend()
plt.show()
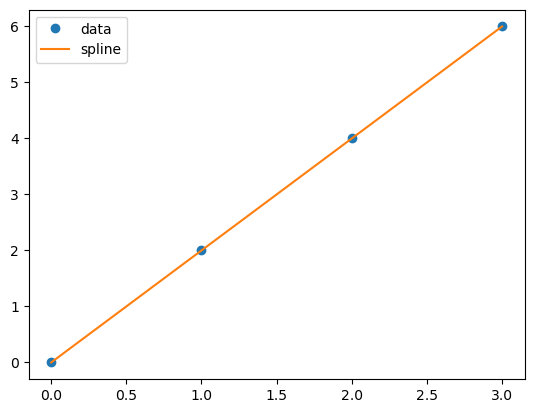
Common extrapolation methods: Linear Extrapolation, Polynomial Extrapolation & Spline Extrapolation with Python Code
Extrapolation is the technique of estimating values outside the range of known data points. Extrapolation can be useful when trying to predict trends in data that extends beyond the available data. However, extrapolation can also be unreliable and can lead to inaccurate results if the data is not well-behaved. Here are three popular extrapolation methods:
Linear Extrapolation with Python Code
What is Linear Extrapolation?
Linear extrapolation is a simple method that extends a straight line beyond the range of known data points. It assumes that the relationship between the known data points is linear and that this relationship continues beyond the range of the data. Linear extrapolation is the easiest method to use, but it can be highly unreliable for data that doesn’t follow a straight line.
Python Code for Linear Extrapolation
Here is an example of linear extrapolation in Python:
import numpy as np
# Example data
x = np.array([0, 1, 2, 3])
y = np.array([0, 2, 4, 6])
# Linear extrapolation function
slope = (y[-1] - y[-2]) / (x[-1] - x[-2])
intercept = y[-1] - slope * x[-1]
x_new = np.array([4, 5])
y_new = slope * x_new + intercept
# Plot original data and extrapolated values
import matplotlib.pyplot as plt
plt.plot(x, y, 'o', label='data')
plt.plot(x_new, y_new, '-', label='linear extrapolation')
plt.legend()
plt.show()
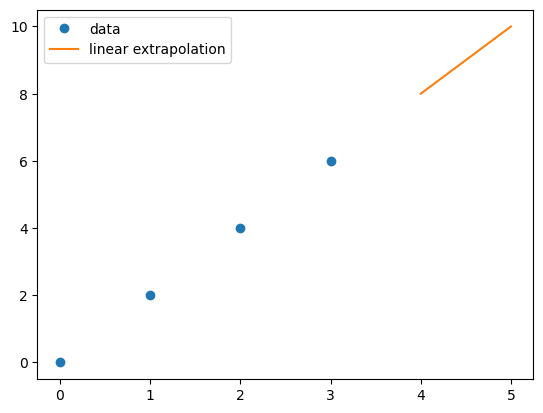
Polynomial Extrapolation with Python Code
What is Polynomial Extrapolation?
Polynomial extrapolation is a method that fits a polynomial curve to the data points and extends the curve beyond the range of the data. It is more accurate than linear extrapolation for data that doesn’t follow a straight line, but it can still be unreliable for highly complex data.
Python Code for Polynomial Extrapolation
Here is an example of polynomial extrapolation in Python:
import numpy as np
# Example data
x = np.array([0, 1, 2, 3])
y = np.array([0, 1, 4, 9])
# Polynomial extrapolation function
p = np.polyfit(x, y, deg=2)
x_new = np.array([3, 4])
y_new = np.polyval(p, x_new)
# Plot original data and extrapolated values
import matplotlib.pyplot as plt
plt.plot(x, y, 'o', label='data')
plt.plot(x_new, y_new, '-', label='polynomial extrapolation')
plt.legend()
plt.show()
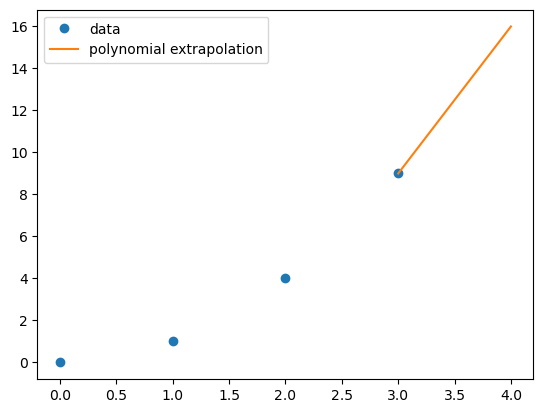
Spline Extrapolation with Python Code
What is Spline Extrapolation?
Spline extrapolation is a method that fits a piecewise polynomial curve to the data points and extends the curve beyond the range of the data. It is the most accurate method for extrapolation and can handle highly complex data, but it can also be the most computationally expensive.
Python Code for Spline Extrapolation
Here is an example of spline extrapolation in Python:
import numpy as np
from scipy.interpolate import splrep, splev
# Example data
x = np.array([0, 1, 2, 3])
y = np.array([0, 2, 4, 6])
# Spline extrapolation function
spl = splrep(x, y, k=1)
x_new = np.array([3, 4])
y_new = splev(x_new, spl, der=0)
# Plot original data and extrapolated values
import matplotlib.pyplot as plt
plt.plot(x, y, 'o', label='data')
plt.plot(x_new, y_new, '-', label='spline extrapolation')
plt.legend()
plt.show()
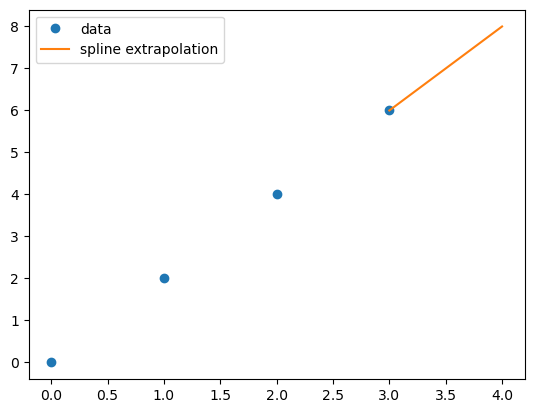
Frequently Asked Questions
What is the difference between interpolation and extrapolation in statistics?
Interpolation is estimating values within the range of existing data, while extrapolation is estimating values outside the range of the data. Interpolation uses observed data to construct a function that can estimate values within the data range, while extrapolation assumes that the underlying pattern in the data continues beyond the range of the data to estimate values beyond what is observed. However, extrapolation can be unreliable because it relies on assumptions about the underlying pattern, and caution should be exercised when using it.
What is an example of interpolation and extrapolation?
An example of interpolation would be estimating the temperature at a time between two observed temperature measurements, while extrapolation would be estimating the temperature outside the range of the observed data. For instance, estimating the temperature at 3pm based on measurements at 12pm and 6pm is interpolation, while estimating the temperature at 9pm based on the same two measurements is extrapolation. Extrapolation can be less reliable than interpolation and requires caution due to its dependence on assumptions.
What is the main difference between interpolation and regression?
The main difference between interpolation and regression is their purpose. Interpolation is used to estimate values within the range of observed data points, while regression is used to model and predict the relationship between variables. Interpolation involves constructing a function or curve that passes through the observed data points, while regression involves fitting a mathematical equation to the observed data points to describe the relationship between the variables.
What is the main difference between extrapolation and regression?
The main difference between extrapolation and regression is that extrapolation involves estimating values outside the range of observed data, while regression is used to model and predict the relationship between variables within the observed data range. Extrapolation relies on assumptions about the continuity of the underlying pattern beyond the observed data, while regression is based on the observed data within the range.
Conclusion
Interpolation and extrapolation both try to extend what we have observed—to what we have not observed but do so in different directions or modes. Interpolation extends to what must have happened between observations, while extrapolation extends to what happens before, after, or beyond observations.
I highly recommend checking out this incredibly informative and engaging professional certificate Training by Google on Coursera:
Google Advanced Data Analytics Professional Certificate
There are 7 Courses in this Professional Certificate that can also be taken separately.
- Foundations of Data Science: Approx. 21 hours to complete. SKILLS YOU WILL GAIN: Sharing Insights With Stakeholders, Effective Written Communication, Asking Effective Questions, Cross-Functional Team Dynamics, and Project Management.
- Get Started with Python: Approx. 25 hours to complete. SKILLS YOU WILL GAIN: Using Comments to Enhance Code Readability, Python Programming, Jupyter Notebook, Data Visualization (DataViz), and Coding.
- Go Beyond the Numbers: Translate Data into Insights: Approx. 28 hours to complete. SKILLS YOU WILL GAIN: Python Programming, Tableau Software, Data Visualization (DataViz), Effective Communication, and Exploratory Data Analysis.
- The Power of Statistics: Approx. 33 hours to complete. SKILLS YOU WILL GAIN: Statistical Analysis, Python Programming, Effective Communication, Statistical Hypothesis Testing, and Probability Distribution.
- Regression Analysis: Simplify Complex Data Relationships: Approx. 28 hours to complete. SKILLS YOU WILL GAIN: Predictive Modelling, Statistical Analysis, Python Programming, Effective Communication, and regression modeling.
- The Nuts and Bolts of Machine Learning: Approx. 33 hours to complete. SKILLS YOU WILL GAIN: Predictive Modelling, Machine Learning, Python Programming, Stack Overflow, and Effective Communication.
- Google Advanced Data Analytics Capstone: Approx. 9 hours to complete. SKILLS YOU WILL GAIN: Executive Summaries, Machine Learning, Python Programming, Technical Interview Preparation, and Data Analysis.
It could be the perfect way to take your skills to the next level! When it comes to investing, there’s no better investment than investing in yourself and your education. Don’t hesitate – go ahead and take the leap. The benefits of learning and self-improvement are immeasurable.
You may also like:
- Extrapolation vs Interpolation: Doesn’t Have To Be Hard
- Top 5 Python programs you must know
- Essential Python Snippets for Data Cleaning and Preparation
- Linear Regression for Beginners: A Simple Introduction
- Linear Regression, heteroskedasticity & myths of transformations
- Bayesian Linear Regression Made Simple with Python Code
- Logistic Regression for Beginners
- Understanding Confidence Interval, Null Hypothesis, and P-Value in Logistic Regression
- Logistic Regression: Concordance Ratio, Somers’ D, and Kendall’s Tau
Check out the table of contents for Product Management and Data Science to explore the topics. Curious about how product managers can utilize Bhagwad Gita’s principles to tackle difficulties? Give this super short book a shot. This will certainly support my work. After all, thanks a ton for visiting this website.